I had to integrate Emma with Ant for my project. I will explain the basic steps involved in doing it. Let’s consider that your directory structure is like this-
Base dir
==Source- Containing the source files.
==Classes – Containing all the class files.
==Test – Containing all the JUNit files.
==Lib – Containing all the jar files needed.
The first task is to set the properties & the class paths. This for our directory structure can be done as:
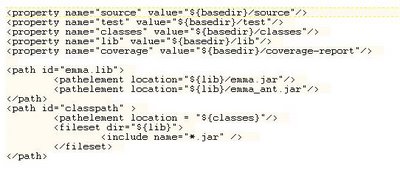
The second task in your ant code is to compile all the file source and test files.
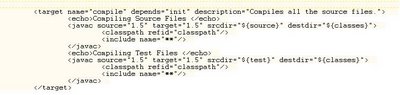
Now we have the class files in the classes directory.The third and the last task is to perform test coverage using emmajava. But before that, emma task should be defined as shown below:
Now we are all set to use emmajava. It can be done as follows:
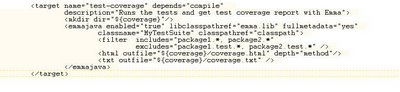
You have to substitute the “MyTestSuite”, with a class that starts your testing. Also include & exclude packages according to your needs. The above code generates both html and txt reports (which are placed in the coverage-report folder that was newly created).
The complete documentation of emmajava can be seen at emma’s site .